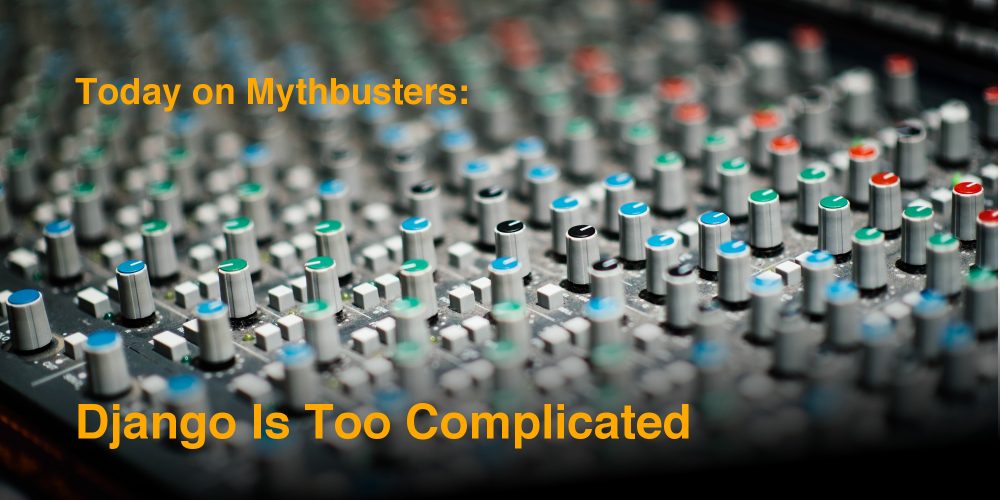
Django is too complicated!
There is a myth out there on the Internet, a myth that needs to be busted.
It’s the idea that Django is too complicated.
And because it is too complicated, it is too difficult to learn for beginners and also unsuited for small applications or microservices.
Where does this idea come from? I’m not 100% sure but I have a theory and the primary suspect is called settings.py
.
Let’s start a Django project to illustrate what I mean. First, we create and activate a virtual environment and install Django:
$ virtualenv venv $ source venv/bin/activate (venv)$ pip install Django ... Successfully installed Django-2.1 pytz-2018.5
After installing Django, we can use the django-admin.py
command which we can use to start our project:
(venv)$ django-admin.py startproject mydjangoproject
Okay, what did that do? It created a directory mydjangoproject
. Let’s have a look at the contents of this directory:
(venv)$ tree mydjangoproject mydjangoproject ├── manage.py └── mydjangoproject ├── __init__.py ├── settings.py ├── urls.py └── wsgi.py
That looks manageable. Until you open settings.py
and get hit by 120 lines of gibberish.
To be fair, about a third of those 120 lines are empty, but there are still 26 distinct settings, some of which are intimidating nested dictionaries. And our app doesn’t even do anything yet!
Compare that to a Hello World app in Flask:
from flask import Flask app = Flask(__name__) @app.route("/") def hello(): return "Hello World!"
Six lines of code and we are done!
However, this comparison is not entirely fair. Without a doubt, Django is a massive framework that makes your life a lot easier with a vast amount of features. But it is entirely up to you to decide which of these features you want to use.
If you do not want to use any of Django’s features, you can use it almost like a microframework.
What, you don’t believe me? Here is a fully functional Hello World app in Django:
$ wc -l *.py 0 __init__.py 3 settings.py 6 urls.py 5 views.py 5 wsgi.py 19 total
That looks a lot more manageable! Most notably, settings.py
shrank to just only three lines:
ROOT_URLCONF = 'mytinydjangoproject.urls' SECRET_KEY = 'fpT6ABCJzwtaWHBXFyzgCybDzYyTxyPdaxEynvA7CwrkVBgAPEJJfie^NB&spxGs' ALLOWED_HOSTS = ['127.0.0.1']
So why does django-admin.py
overwhelm you with this giant settings file?
The explanation might lie in the Zen of Python, which says:
Explicit is better than implicit.
Instead of hiding some default settings somewhere in its code, Django generates a settings file with sensible defaults as part of your project. If those defaults don’t suit your use-case, you are free to tailor them to your needs.
With our minimal settings file, you won’t benefit from the majority of Django’s features: database abstraction and a powerful automatic admin interface, template loading and rendering, user management, static file handling, to name a few.
You could start with an empty settings file and add the features you need, but I find it easier to remove the parts I don’t need from the settings file generated by Django.
The inconvenient truth is that web development, in general, is a pretty complicated affair. Django solves many problems that you might not even be aware of.
Take CSRF protection, for example. It is enabled by default when you start a new project and can be a stumbling block, especially if you are a beginner. You have to remember to add the {% csrf_token %}
to your forms and might grow some grey hairs before you figure out how to get your AJAX requests to work. I still think that enabling CSRF protection by default makes perfect sense. A novice programmer might not know that CSRF exists, how a CSRF attack works, and much less how to protect against it.
So what’s the final verdict? Is Django too complicated?
As you might have guessed, my answer is No.
If you want to share your opinion, leave a comment below, drop me an email or get in touch on Twitter.
Header image by chuttersnap
imo, below are the reasons django is really complicated ( many other web frameworks are) –
1. django overdid DRY.
2. you can do same things multiple ways. see pep 20 – There should be one– and preferably only one –obvious way to do it.
So newbies follows django official tutorials successfully but when they try to do hack it for their needs, it becomes big mess.
Thanks for sharing your thoughts! I think your second point kind of contradicts the first. If there is only one way to do it, wouldn’t that imply even more DRY?
That being said, I agree with your first point when it comes to Generic Class-Based views. It is often more complicated to figure out the class hierarchy and which method to overwrite than just creating a view from scratch.
But I think in many other areas Django hits the sweet-spot between over-generalization and forcing the user to reinvent the wheel.
Nothing seems complicated if you know how to use it already. Beginners coming in don’t have problems because they’re dumb, they have problems because they have expectations about how to do something and rather than seeing something that’s slightly different and adjusting, what they instead find is that in order to do something as simple as render a user-uploaded avatar on a form where they can change it, they have to read documentation for three days, and even after that, they’re hitting up stackoverflow because it’s impossible to turn three days’ worth of documentation into something concise that works.
Sorry, I disagree… Django is crazy difficult! I am a new programmer, completely self-taught. I already built a full-featured, large inventory management program that is currently in use at a large company. It was a great project and fun. I built it using flask. Now I am tackling a new project and thought I would try django. WOW, it has been an epic nightmare. Weeks and weeks of reading docs, google, SO, just to figure out something that I could code in 10 minutes using flask. Im about 50% of the way thorugh in this new project and about to throw in the towel and start all over using good ‘ol comfortable flask 🙁
It’s is difficult the amount of over head you need to know before you can make something is insane.
“You need a db… ”
– for what I I just want to make a hello world home page
“you need to make a new app”
– but thats what i thought I was already doing.
“you have an incorrect url pattern”
– i spent a good hour figuring it out and I still don’t know how it works
“you need to run migrations”
… why I just want to make a hello world page.
The difference in flask is I can build on something small, learning a piece at a time.
Django’s Philosophy seems to be
step 1: be a django expert
step 2: develop in django
It has gotten to the point that django seems to get in the way of my ideas rather then being a useful too to help facilitate them.
That’s the point I tried to get across with this post: you can build much simpler applications with Django, it’s just not obvious how. Once you want to use a database and know (why) you need migrations, Django provides a well-integrated package, where you have to piece everything together with other frameworks.